Whether you are new to jQuery or not, you must have come across a situation where you had to check if an element is hidden in jQuery. While this might sound like an easy task, it can get tricky. Don’t worry, you can check if an element is hidden in jQuery using the simple ways shown below.
jQuery’s inbuilt selector “:hidden” can be handy when you want to check if an element is hidden on the page. The code for the same is as follows
// This checks if the element has display:none.
$(element).is(":hidden");
The above selector would check if the element is “hidden” in the sense that whether the element has display:none CSS property to it. Please note that this would not return true for an element that has visibility:hidden CSS property set to it. As per jQuery’s documentation the Elements with visibility: hidden or opacity: 0 are considered to be visible as they still take up space on the layout.
The “:hidden” selector would return true only for the elements that suffice the following conditions:
- They have a CSS display:none value to it.
- They are input elements with type=”hidden”.
- Their width and height are set to 0.
- A parent element is hidden, so the element is not shown on the page.
Here is an example of the output I was getting while trying out the :hidden selector in jQuery. In the code you can see that the element with CSS property visibility:hidden gives a false when we try to check if it is hidden. Whereas, the element with display:none returns true.
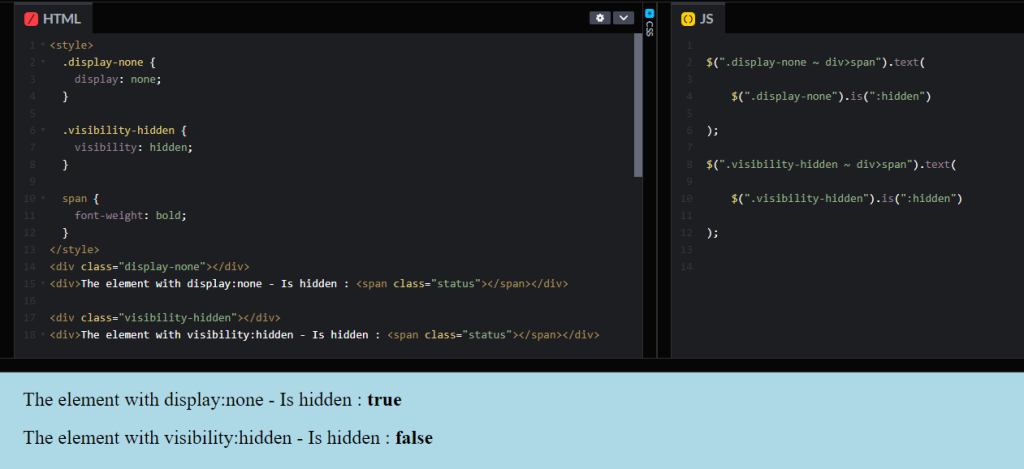
While jQuery’s “:hidden” selector does a good job in identifying “certain” types of hidden elements on the page, it still leaves out elements that are visibly hidden but take up space on the page. Elements with CSS property visibility:hidden would still not be recognized as hidden by jQuery. In order to include elements that have visibility:hidden too in your search for a hidden element, you can use the following code.
if ($(element).is(':hidden') || $(element).css("visibility") == "hidden") {
// 'element' is truly hidden
}
The above code would check if the element is considered to be hidden by jQuery and also if the element is visibly hidden using the CSS visibility:hidden property. You can use this if you would like to cover elements that are both hidden and does not take up space (display:none), and those elements too which are hidden but takes up space(visibility:hidden). It would also cover the elements that are of input type hidden, that have width and height of 0, and also those which are hidden because their parent element is hidden.