It is very easy to check if a checkbox is checked in jQuery using the .is() or .prop() method of jQuery combined with the ‘:checked’ selector. There are other ways too like checking for the attribute checked value, however, they aren’t very reliable. The easiest and most stable way to check if checkbox is checked in jQuery is to use the .is(‘:checked’) method or the .prop(‘checked’) method. Let us take a look at how to do so using both the methods along with examples.
Solutions to check if checkbox is checked in jQuery
There are 2 solutions to check if checkbox is checked in jQuery.
- Solution 1 : Using the is() jQuery method
- Solution 2 : Using the prop() jQuery method
Let us take a look at both of these methods with examples.
Solution 1: Using the .is() jQuery method to check if checkbox is checked
As I mentioned earlier, one of the effective ways to check if checkbox is checked is to use the .is() jquery method along with the ‘:checked‘ selector. Let us take a look at how to do it.
Example showing how to check if checkbox is checked in jQuery using .is(‘:checked’)
In the example below we’ll see how to check if checkbox is checked on button click using jQuery.
The jQuery Code
function statusCheck() {
let status = $('#colorcheckbox').is(':checked');
console.log(status);
}
The HTML code
<input type="checkbox" id="colorcheckbox" name="color" value="red"/> Red
<button onclick="statusCheck()">Check</button>
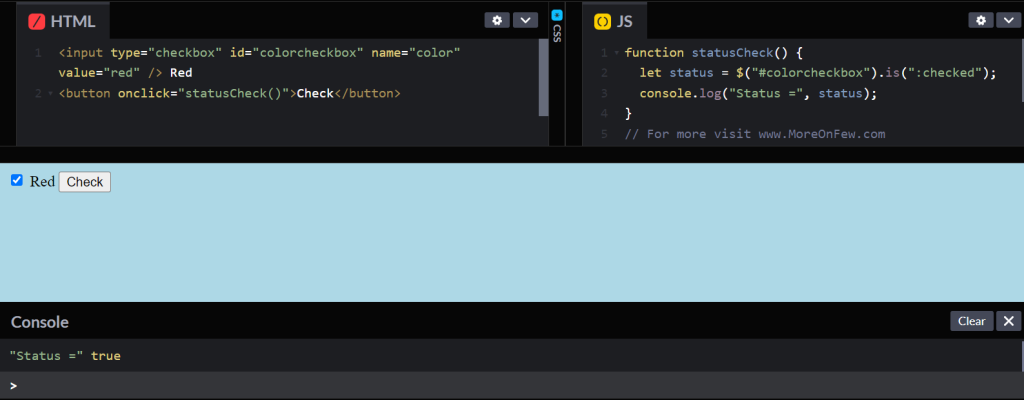
As you can see in the code above, we are using the $(element).is(‘:checked’) method to find out if the checkbox is checked. When the checkbox gets checked, we get a boolean value of true in return. Whereas, if the checkbox is unchecked, it would return a boolean value of false. Since the .is(‘:checked’) returns a boolean value in return, we can use it to write our conditional statements. For example:
if ($('#colorcheckbox').is(':checked')) {
// some code here that executes only when the checkbox is checked
}
//OR
const CheckboxStatus = $('#colorcheckbox').is(':checked');
if (CheckboxStatus) {
// code here
}
Solution 2: Using the .prop() jQuery method to check if checkbox is checked
The second solution to check if checkbox is checked in jQuery is to use the .prop() method in jQuery. This is very similar to the previous solution of using .is() method. However, instead of using .is(), we would be using the .prop() method. Let us take a look at an example.
Example showing how to check if checkbox is checked in jQuery using the .prop() method
function statusCheck() {
let status = $("#colorcheckbox").prop("checked"); // use .prop() method
console.log("Status =", status);
}
In the code above you can notice that we have used the .prop() method instead of the .is() method. Also, if you notice carefully, we have used just “checked” instead of the “:checked”.
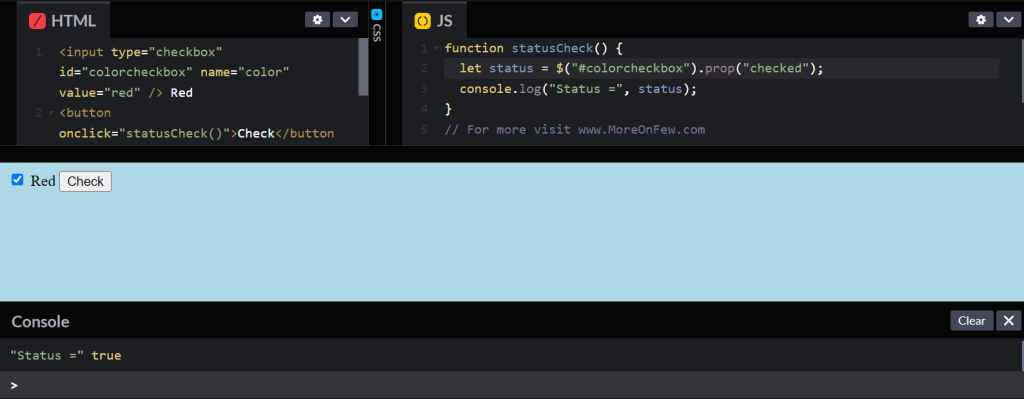
Similar to the .is(‘:checked’) method, the .prop(‘checked’) method too returns a boolean value of true or false. Hence, we can use it to write conditional statements too.
To conclude, you can see that we can either use the .is(‘:checked’) method or we can use the .prop(‘checked’) method to easily know if a checkbox is checked in jQuery. JQuery has many such methods and selectors which when used correctly, makes it easy for us to access the DOM elements or their attributes and values. Hope you got the answer to your query. Check out our other jQuery tutorials and articles too.